CADViewer, with it’s flexible design and use of standard toolkits, can be integrated with any Database Management Application and be used with a multitude of custom data-driven applications.
This Tutorial
This tutorial builds on top of the CADViewer tutorials Guide - Automate Hotspots and Guide - Add & Edit Hotspots where a base CAD drawing containing space poloygons on a designated layer been made interactive in CADViewer and then subsequently, interactive content has been added to the CAD drawing.
This tutorial will outline various ways of:
- 1: Highlight all Space Objects.
- 2: Highlight a single Space Object ID.
- 3: Highlight a given Space Type.
- 4: Control style to highlight Space Object borders.
- 5: Add Styled Text to Space Objects.
- 6: Use the API to apply Hatching to Space Objects.
- 7: Clear all Highlights from Space Objects.
- 8: Use the API to pull content from Space Objects.
- 9: Set up User Control of Content for the Pop-Up Space Modals.
- 10: Hide Pop-Up Space Modals and replace with custom code.
The steps outlined below can be tested in our Download versions of CADViewer. Furhermore, use our online reference sample CADViewer_space_objects_canvas_API_640.html as the base for this tutorial
1: Highlight All Space Objects.
The CADViewer API provides an API call to highlight a space based on a CSS style object and a Space Object ID.
The process to highlight all, is to first extract a list with all Space objects using the API method: function cvjs_getSpaceObjectIdList() and then loop over the list of spaces and apply the CSS style object colortype to each Space using function cvjs_highlightSpace():
function highlight_all_spaces(){
// selectedColor is set through color selector in Demo
var colortype = {
fill: selectedColor,
"fill-opacity": "0.8",
stroke: selectedColor,
'stroke-width': 1,
'stroke-opacity': "1",
'stroke-linejoin': 'round'
};
var spaceObjectIds = cvjs_getSpaceObjectIdList();
for (spc in spaceObjectIds){
var rmid = spaceObjectIds[spc];
cvjs_highlightSpace(rmid, colortype);
}
}
2: Highlight a single Space Object ID
The CADViewer API provides an API call to highlight a space based on a CSS style object and a Space Object ID, and highlight using: function cvjs_highlightSpace(). In the online sample, a check has been added, so only an existing SpaceID is highlighted.
function highlight_space_id(){
// selectedColor is set through color selector in Demo
var spaceid = $('#image_ID').val();
var colortype = {
fill: selectedColor,
"fill-opacity": "0.8",
stroke: selectedColor,
'stroke-width': 1,
'stroke-opacity': "1",
'stroke-linejoin': 'round'
};
var spaceObjectIds = cvjs_getSpaceObjectIdList();
for (spc in spaceObjectIds){
console.log(spaceid+" "+spaceObjectIds[spc]+" "+(spaceid.indexOf(spaceObjectIds[spc])==0)+" "+((spaceid.length == spaceObjectIds[spc].length)));
if (spaceid.indexOf(spaceObjectIds[spc])==0 && (spaceid.length == spaceObjectIds[spc].length) )
cvjs_highlightSpace(spaceObjectIds[spc], colortype);
}
}
3: Highlight a given Space Type
The process to highlight all given Space Types, is to first extract a list with all Space objects using the API method: function cvjs_getSpaceObjectIdList() and then loop over the list of spaces, check if space has a given type using, function cvjs_getSpaceObjectTypefromId(), and apply the CSS style object colortype to each Space using function cvjs_highlightSpace():
function highlight_space_type(){
// selectedColor is set through color selector in Demo
var type = $('#image_Type').val();
var colortype = {
fill: selectedColor,
"fill-opacity": "0.8",
stroke: '#fe50d9',
'stroke-width': 1,
'stroke-opacity': "1",
'stroke-linejoin': 'round'
};
var spaceObjectIds = cvjs_getSpaceObjectIdList();
for (spc in spaceObjectIds){
var spaceType = cvjs_getSpaceObjectTypefromId(spaceObjectIds[spc]);
var vqid = spaceObjectIds[spc];
console.log(spc+" "+ vqid + " "+spaceType+ " "+spaceType.indexOf("Device"));
if (spaceType.indexOf(type)==0)
cvjs_highlightSpace(vqid, colortype);
}
}
4: Control style to highlight Space Object Borders
The CADViewer API provides an API call to highlight a space based on a CSS style object and a Space Object ID.
The process to highlight Space Borders, is to first extract a list with all Space objects using the API method: function cvjs_getSpaceObjectIdList() and then loop over the list of spaces and apply the CSS style object colortype, with border style, to each Space using function cvjs_highlightSpace():
function highlight_all_borders(){
// selectedColor is set through color selector in Demo
var colortype = {
fill: '#fff',
"fill-opacity": 0.01,
stroke: selectedColor,
'stroke-width': 3.0,
'stroke-opacity': 1,
'stroke-linejoin': 'round'
};
var spaceObjectIds = cvjs_getSpaceObjectIdList();
for (spc in spaceObjectIds){
var rmid = spaceObjectIds[spc];
cvjs_highlightSpace(rmid, colortype);
}
}
5: Add Styled Text to Space Objects
Text Layer - declaration and initialization
Styled text on a Space Object needs to be placed on a separate text layer. This layer should be declared globally.
var textLayer1;
The layer needs to be initialized and cleared for usage through the API command cvjs_clearLayer() before usage. A good location for this, is after the drawing is loaded, so therefore inside the call-callback method cvjs_OnLoadEnd().
function cvjs_OnLoadEnd() {
// ..
textLayer1 = cvjs_clearLayer(textLayer1);
//..
}
API Command to add multiple lines of text inside Space Object
The function cvjs_AddTextOnSpaceObject(), adds text on a textLayer based on the Space ID. The user must set arrays containing styles, sizes, color and the text objects. The method also povide controls to center and clip text content within the Space Object.
/**
* Add multiple lines of text, individually formatted and styled, inside a Space Object
* @param {string} txtLayer - layer to apply the text
* @param {string} Id - Id of the graphical object in which to place the text
* @param {float} leftScale - distance from the left border of Space Object, value between 0 and 1
* @param {array} textStringArr - Array with the lines of text
* @param {array} textStyleArr - Array with textstyle of text lines, formattet as a java script object with css style elements, predefined is: text_style_arial_11pt_bold , text_style_arial_9pt_normal, text_style_dialog
* @param {array} scaleTextArr - Array with relative scale of text lines in relation to space object height, value between 0 and 1. If 0, using font-size in font object as is.
* @param {array} hexColorTextArr - Array of color of text lines in hex form, for example: #AA00AA
* @param {boolean} clipping - true if clipping of text inside of Space Object, false if text to cross Space Object borders
* @param {boolean} centering - true if centering of text inside of Space Object, false is default
*/
function cvjs_AddTextOnSpaceObject(txtLayer, Id, leftScale, textStringArr, textStyleArr, scaleTextArr, hexColorTextArr, clipping, centering){};
Text Styles
A typical text style element used with cvjs_AddTextOnSpaceObject() is a JSON variable containing font styling and settings. Below are a few samples:
/* text style for adding text into Space Objects */
var text_style_arial_11pt_bold = {
'font-family': "Arial",
'font-size': "11pt",
'font-weight': "bold",
'font-style': "none",
'margin': 0,
'cursor': "pointer",
'text-align': "left",
'z-index': 1980,
'opacity': 0.5
};
/* text style for adding text into Space Objects */
var text_style_arial_9pt_normal = {
'font-family': "Arial",
'font-size': "9pt",
'font-weight': "normal",
'font-style': "none",
'margin': 0,
'cursor': "pointer",
'text-align': "left",
'z-index': 1980,
'opacity': 1
};
/* text style for adding text into Space Objects */
var text_style_arial_14pt_normal = {
'font-family': "Arial",
'font-size': "14pt",
'font-weight': "normal",
'font-style': "none",
'margin': 0,
'cursor': "pointer",
'text-align': "left",
'z-index': 1980,
'opacity': 1
};
/* text style for adding text into Space Objects */
var text_style_dialog = {
'font-family': "Dialog",
'font-size': "9pt",
'font-weight': "normal",
'font-style': "italic",
'margin': 0,
'cursor': "pointer",
'text-align': "left",
'z-index': 1980,
'opacity': 1
};
Sample with Multiple Lines of Text
The process to add multiple lines of styled text to Space Object, is to first extract a list with all Space objects using the API method: function cvjs_getSpaceObjectIdList() and then loop over the list of spaces and apply the text to each Space using function cvjs_AddTextOnSpaceObject().
// This is the function that illustrates how to label text inside room polygons
function customAddTextToSpaces(){
// I am making an API call to the function cvjs_getSpaceObjectIdList()
// this will give me an array with IDs of all Spaces in the drawing
var spaceObjectIds = cvjs_getSpaceObjectIdList();
var i=0;
var textString ;
var textStyles ;
var scaleText ;
var hexColorText;
for (var spc in spaceObjectIds)
{
//console.log(spaceObjectIds[spc]+" "+i);
if ((i % 3) ==0){
textString = new Array("Custom \u2728", "settings \u2728", "\u2764\u2728\u267B");
textStyles = new Array(text_style_arial_9pt_normal, text_style_arial_11pt_bold, text_style_dialog);
scaleText = new Array(0.15, 0.2, 0.15 );
hexColorText = new Array("#AB5500", "#66D200", "#0088DF");
// here we clip the roomlables so they are inside the room polygon
cvjs_AddTextOnSpaceObject(textLayer1, spaceObjectIds[spc], 0.05, textString, textStyles, scaleText, hexColorText, true, false);
}
else{
if ((i % 3) == 1){
textString = new Array('Unicode:\uf083\uf185\u2728', 'of custom text');
textStyles = new Array(FontAwesome_9pt_normal, text_style_dialog);
scaleText = new Array(0.15, 0.15 );
hexColorText = new Array("#00D2AA", "#AB0055");
// here we clip the roomlables so they are inside the room polygon
cvjs_AddTextOnSpaceObject(textLayer1, spaceObjectIds[spc], 0.1, textString, textStyles, scaleText, hexColorText, true, false);
}
else{
textString = new Array("\uf028");
textStyles = new Array(FontAwesome_9pt_normal);
scaleText = new Array( "0.5" );
hexColorText = new Array("#AAAAAA");
//var hexColorText = new Array("#00AADF");
// here we clip the roomlables so they are inside the room polygon, we center object
cvjs_AddTextOnSpaceObject(textLayer1, spaceObjectIds[spc], 0, textString, textStyles, scaleText, hexColorText, true, true);
}
}
i++;
}
Sample with single line Centered Text
The process to add a single line of styled text centered on a Space Object, is to first extract a list with all Space objects using the API method: function cvjs_getSpaceObjectIdList() and then loop over the list of spaces and apply the text to each Space using function cvjs_AddTextOnSpaceObject().
Note: Left scale factor, leftScale, is set to -0.3 to move text 30% to the left of space object center location in x-axis direction.
function customAddIDToSpaces() {
// First an API call to the function cvjs_getSpaceObjectIdList()
// this will me an array with IDs of all Spaces in the drawing
var spaceObjectIds = cvjs_getSpaceObjectIdList();
var textString;
var textStyles;
var scaleText;
var hexColorText;
for (var spc in spaceObjectIds) {
textString = new Array(spaceObjectIds[spc]);
textStyles = new Array(text_style_arial_14pt_normal);
scaleText = new Array("0"); // scale in relation to object height, if zero use font-size as is.
hexColorText = new Array("#000");
cvjs_AddTextOnSpaceObject(textLayer1, spaceObjectIds[spc], -0.3, textString, textStyles, scaleText, hexColorText, true, true);
}
}
Clear the Text Layer
Whenever the Text Layer needs to be cleared, simply call the API command cvjs_clearLayer():
textLayer1 = cvjs_clearLayer(textLayer1);
}
6: Use the API to apply Hatching to Space Objects
The function function cvjs_hatchSpace(), hatches a Space Object based on the Space ID, hatchtype color and opacity. The method supports 14 different types of hatches.
/**
* Apply a predefined pattern on a graphical Space Object
* @param {string} rmid - Id of the Space Object object to add hatches
* @param {string} graphicalPattern - name of the hatch pattern, from a predefined list of patterns:
* "pattern_45degree_standard"
* "pattern_45degree_fine"
* "pattern_45degree_wide"
* "pattern_90degree_standard"
* "pattern_90degree_fine"
* "pattern_90degree_wide"
* "pattern_135degree_standard"
* "pattern_135degree_fine"
* "pattern_135degree_wide"
* "pattern_0degree_standard"
* "pattern_0degree_fine"
* "pattern_0degree_wide"
* "pattern_45degree_crosshatch_standard"
* "pattern_45degree_crosshatch_fine"
* @param {string} colorHex - color of hatch in hex form, for example: #FF0000
* @param {float} fillOpacity - fill opacity of pattern, value between 0 and 1
*/
The process to hatch Space Object, is to first extract a list with all Space objects using the API method: function cvjs_getSpaceObjectIdList() and then loop over the list of spaces and apply the hatch method each Space using function cvjs_hatchSpace().
// hatch spaces
var hatchtype = 0;
function customHatchSpaces(){
// I am making an API call to the function cadviewer.cvjs_getSpaceObjectIdList()
// this will give me an array with IDs of all Spaces in the drawing
var spaceObjectIds = cvjs_getSpaceObjectIdList();
for (var spc in spaceObjectIds)
{
hatchtype++;
if (hatchtype >4) hatchtype=1;
if (hatchtype == 1) cvjs_hatchSpace(spaceObjectIds[spc], "pattern_45degree_crosshatch_fine", "#550055" , "0.5");
if (hatchtype == 2) cvjs_hatchSpace(spaceObjectIds[spc], "pattern_45degree_standard", "#AA2200" , "0.5");
if (hatchtype == 3) cvjs_hatchSpace(spaceObjectIds[spc], "pattern_135degree_wide", "#0055BB" , "0.5");
if (hatchtype == 4) cvjs_hatchSpace(spaceObjectIds[spc], "pattern_90degree_wide", "#220088" , "0.5");
}
};
7: Clear all Highlights from Space Objects
When clearing CADViewer for highlights, two different objects should be cleared:
- 1: The back-end layer that highlights are drawn upon, is cleard through the API call: function cvjs_clearSpaceLayer()
- 2: Any specific layers used for text decorations, needs to be cleared and returned using: function cvjs_clearLayer()
// in the demo sample, text decorations in Space Objects are done on the layer textLayer1.
function clear_space_highlight(){
cvjs_clearSpaceLayer();
textLayer1 = cvjs_clearLayer(textLayer1);
}
8: Use the API to pull content from Space Objects
The function cvjs_returnAllSpaceObjects, returns the content of all Space Objects as JSON object. This JSON object can then be parsed for specific values. In the sample case below, Spaces and their calculated Area are extracted and listed.
////////// FETCH ALL SPACE OBJECTS
function display_all_objects(){
/* Return a JSON structure with all Space Object content, each entry is of the form: <br>
* SpaceObjects :[ { "path": path, <br>
* "tags": tags, <br>
* "node": node, <br>
* "area": area, <br>
* "outerhtml": outerHTML, <br>
* "occupancy": occupancy, <br>
* "name": name, <br>
* "type": type, <br>
* "id": id, <br>
* "defaultcolor": defaultcolor, <br>
* "layer": layer, <br>
* "group": group, <br>
* "linked": linked, <br>
* "attributes": attributes, <br>
* "attributeStatus": attributeStatus, <br>
* "displaySpaceObjects": displaySpaceObjects, <br>
* "translate_x": translate_x, <br>
* "translate_y": translate_y, <br>
* "scale_x": scale_x ,<br>
* "scale_y": scale_y ,<br>
* "rotate": rotate, <br>
* "transform": transform, <br>
* "svgx": svgx, <br>
* "svgy": svgx, <br>
* "dwgx": dwgx, <br>
* "dwgy": dwgy } <br>
* @param {string} spaceID - Id of the Space Object to return
* @return {Object} jsonSpaceObject - Object with all space objects content
*/
// get json obhect with all spaces processed from drawing
var allSpaceObjects = cvjs_returnAllSpaceObjects();
var myString = "";
for (var spc in allSpaceObjects.SpaceObjects){
console.log(spc);
myString += "("+allSpaceObjects.SpaceObjects[spc].id+", "+allSpaceObjects.SpaceObjects[spc].area+")";
}
window.alert("The spaces with area (id,area): "+myString);
}
////////// HIGHLIGHT METHODS START
9: Set up User Controls for the Pop-Up Space Modals
CADViewer, enables application programmers the ability to catch a call-back when an object is clicked, and dynamically set the content of the popup modal. This allows application programmers to interact with a database or back-end and serve object-specific content back to the pop-up modal on the CADViewer canvas.
Please see the detailed documentation in the section Dynamic Modals for Highlight. And please read the section Custom Modals for Highlight on the base set-up of modals.
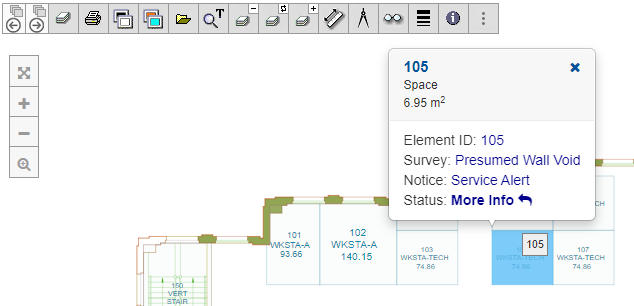
10: Hide Custom Pop-Up Space Modals
Application programmerscan take control over the Pop-Up modals, hide the standard CADViewer Modals and replace with their own interface, please do read the following section Suppress/Exchange Highlight Modals.